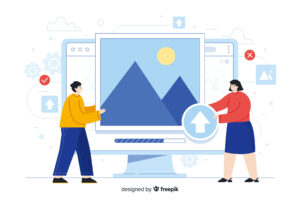
CSSだけで簡単にトップに戻るボタンを作る方法
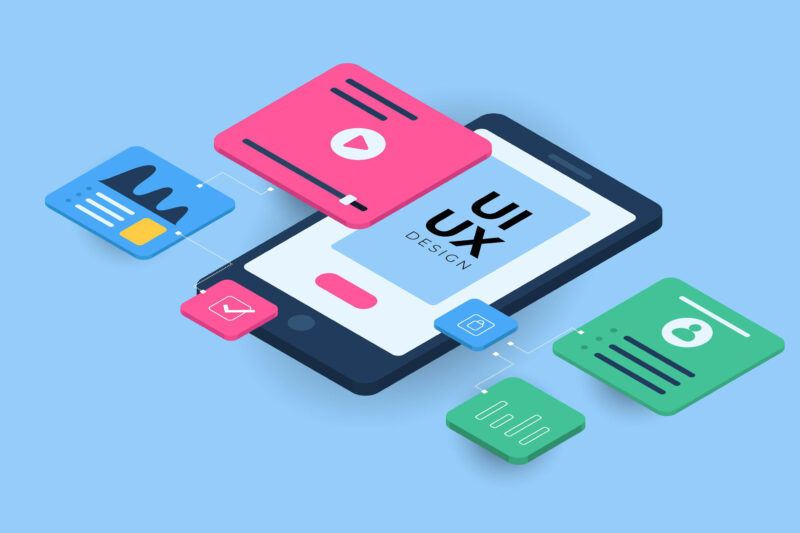
訪れたユーザーにとってストレスなくサイトを閲覧したもらうため、トップに戻るボタンはとても重要です。そのトップに戻るボタンの作り方や右下に固定させたり、スクロールの途中で表示する方法を紹介したいと思います。
[rtoc_mokuji title=”” title_display=”” heading=”” list_h2_type=”” list_h3_type=”” display=”” frame_design=”” animation=””]
基本的なデザイン
See the Pen Untitled by teru (@teru241546) on CodePen.
上記がよく見るボタンのデザインかと思います。ボタンの位置は右下、大きさは48px x 48pxくらいで配色は背景とテキストのコントラストがはっきりしたものが良いですね。
次にコードの解説をしていきます。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge"/>
<title>DEMO</title>
<link rel ="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/css/all.min.css">
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<a href="#"></a>
</body>
</html>
html {
scroll-behavior: smooth;
}
a {
position: fixed;
bottom: 24px;
right: 24px;
width: 48px;
height: 48px;
text-decoration: none;
background-color: #6002EE;
display: block;
text-align: center;
border-radius: 24px;
}
a::before {
content: '\f062';
font-family: 'Font Awesome 6 Free';
font-size: 30px;
font-weight: 900;
color: #fff;
line-height: 48px;
}
HTMLではaタグを使用、属性にhref=”#”と記述することでクリックされたらトップまで戻るしくみになっています。
</head>の上にはFont AwesomeのCDNコードを貼り付け、Font Awesomeのアイコンを使用できるようにしています。
このFont Awesomeを使うことでいろんなアイコンを使用することができます。詳しい使用方法などはこちらの記事を参考にしてみてください。
CSSではaタグに外の形や背景色、a::beforeで中の矢印を装飾しました。aタグにposition: fixed;で要素を浮かし、bottom、rightで右下からちょっと余白を作るぐらいに位置調整させてみました。
そしてhtmlタグにscroll-behavior: smooth;と記述してクリックされた時の戻るスピードをスムーズにすることができますが、細かいスピードの調整まではできません。
実際にどのような感じで戻るのか下記のデモページからご覧ください。
DEMOボタンのaタグを記述する場所は個人的にはfooterの上あたりがいいかなと思っています。
いろんなデザイン
ボタンデザインのサンプルコード一覧です。
- ボタンに影をつける
See the Pen TOPに戻るボタン by teru (@teru241546) on CodePen.
- スタイリッシュな感じ
See the Pen Untitled by teru (@teru241546) on CodePen.
- すりガラス風
See the Pen フォーク by teru (@teru241546) on CodePen.
スクロールの途中からフワッと出てくるようにする
サイトでよく見るスクロールの途中からボタンを表示させるにはJavaScriptを使用していきます。
よくわからない方でもコードをまるっとコピペしてもらえば使用できますので試してみてください。
HTMLのコード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge"/>
<title>DEMO</title>
<link rel ="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/css/all.min.css">
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<header>header</header>
<main>
<section class="section1">section1</section>
<section class="section2">section2</section>
<section class="section3">section3</section>
<section class="section4">section4</section>
</main>
<a href="#" id="page-top"></a>
<footer>footer</footer>
<script src="js/main.js"></script>
</body>
</html>
CSSのコード
html {
scroll-behavior: smooth;
}
body {
margin: 0 ;
padding: 0;
}
header,main,footer {
max-width: 800px;
margin: 0 auto;
text-align: center;
font-size: 30px;
font-weight: 700;
}
header {
height: 120px;
background-color: #333;
color: #fff;
line-height: 120px;
}
section {
height: 1000px;
color: #fff;
line-height: 1000px;
}
.section1 {
background: #7E44F2;
}
.section2 {
background: #6115EE;
}
.section3 {
background: #540FE8;
}
.section4 {
background: #3C00E0;
}
footer {
height: 400px;
background-color: #333;
color: #fff;
line-height: 400px;
}
/* ここからボタンのデザイン */
a {
position: fixed;
bottom: 24px;
right: 24px;
width: 48px;
height: 48px;
text-decoration: none;
background-color: #6002EE;
display: block;
text-align: center;
border-radius: 24px;
opacity: 0;
transition: opacity 0.4s ease-in;
}
a::before {
content: '\f062';
font-family: 'Font Awesome 6 Free';
font-size: 30px;
font-weight: 900;
color: #fff;
line-height: 48px;
}
/* fadeinクラスがついた時だけ表示する */
a.fadein {
opacity: 1;
}
/* ここまでボタンのデザイン */
JavaScriptのコード
window.addEventListener('scroll',() => {
const pageTop = document.getElementById('page-top'); //トップに戻るボタンのidを取得
if(window.pageYOffset >= 400) { //上から400px以上スクロールしたら
pageTop.classList.add('fadein'); //aタグにfadeinクラスを与える
} else {
pageTop.classList.remove('fadein');//aタグからfadeinクラスをはずす
}
});
JavaScriptでは単純に上から400px地点でclassをつけたりはずしたりするだけのトリガーを作りました。
CSSではあらかじめaタグはopacity: 0;として表示を消しときます。a.fadeinでaタグにfadeinクラスがついた時だけ表示するようにしました。
もしよければ試してみてください