【コピペOK】CSSだけでおしゃれな動きをするハンバーガーメニューを作る
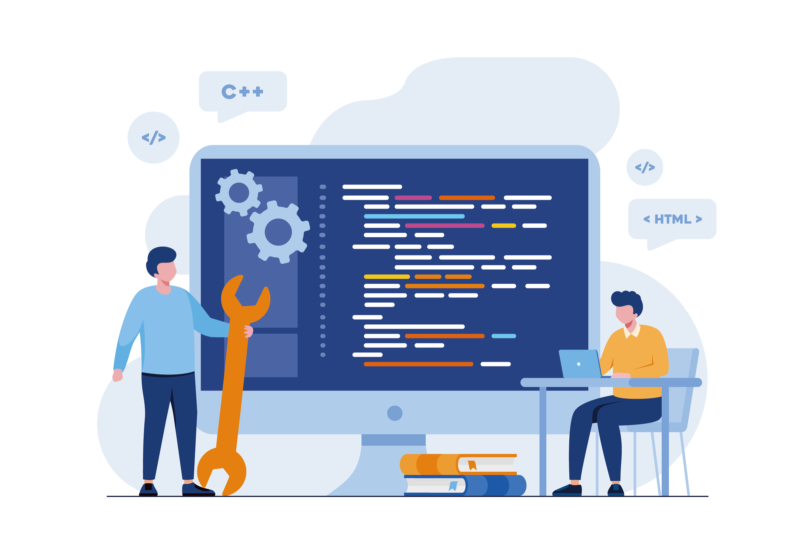
スマートフォンサイトで画面の上の方によくある
上記のような形をしたアイコンをハンバーガーメニューと呼びます。
しかし、これを最初から実装するのはちょっと面倒です。
今回はCSSだけで簡単に作れるハンバーガーメニューを紹介していきたいと思います。気に入ったらコピペしていってください。
ハンバーガーメニューとは
ハンバーガーメニューは横3本線の見た目がハンバーガーに似ていることからその名で呼ばれるようになりました。別名ハンバーガーアイコンとかドロワーメニューとも呼ばれたりします。
このアイコンをクリックすることで必要な時にメニューを表示させたり閉じたりすることができ、スマホなどの小さな画面ではスペースを有効活用するためによく使われています。
CSSだけを使ったハンバーガーメニュー
JavaScriptを使わずCSSだけでハンバーガーメニューを実装します。ポイントはクリック判定にcheckboxを使用するところです。
<body>
<header>
<div class="logo">LOGO</div>
<input type="checkbox" id="checkbox" class="checkbox">
<label for="checkbox" class="hamburger">
<span class="bar bar-top"></span>
<span class="bar bar-middle"></span>
<span class="bar bar-bottom"></span>
</label>
<nav class="nav-menu">
<ul>
<li><a href="#">menu1</a></li>
<li><a href="#">menu2</a></li>
<li><a href="#">menu3</a></li>
<li><a href="#">menu4</a></li>
</ul>
</nav>
</header>
<main></main>
</body>
/* リセットCSS */
html, body, div, span, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
abbr, address, cite, code,
del, dfn, em, img, ins, kbd, q, samp,
small, strong, sub, sup, var,
b, i,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, figcaption, figure,
footer, header, hgroup, menu, nav, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
outline: 0;
font-size: 100%;
vertical-align: bottom;
background: transparent;
list-style: none;
}
body {
overflow-x: hidden;
}
*,
*::before,
*::after {
box-sizing: border-box;
}
a {
display: block;
color: inherit;
text-decoration: none;
font: inherit;
}
/* ここまでリセットCSS */
body {
font-family: "Arial", sans-serif;
color: #555;
font-feature-settings: "palt";
}
html {
scroll-behavior: smooth;
}
header {
position: fixed;
height: 80px;
width: 100%;
padding: 0 20px;
background: #fff;
display: flex;
justify-content: space-between;
align-items: center;
}
.logo {
font-size: 32px;
}
.hamburger {
width: 60px;
height: 60px;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
cursor: pointer;
}
.hamburger .bar {
width: 40px;
height: 3px;
background: #333;
border-radius: 2px;
display: block;
position: absolute;
transition: all 0.3s;
}
.hamburger .bar-top {
transform: translate(0, -12px);
}
.hamburger .bar-bottom {
transform: translate(0, 12px);
}
.checkbox:checked ~ .hamburger .bar-middle {
opacity: 0;
}
.checkbox:checked ~ .hamburger .bar-top {
transform: translate(0, 0) rotate(45deg);
}
.checkbox:checked ~ .hamburger .bar-bottom {
transform: translate(0, 0) rotate(-45deg);
}
.checkbox {
display: none;
}
.nav-menu {
position: fixed;
width: 280px;
height: 100%;
background: #000;
opacity: 0.8;
color: #fff;
top: 0;
left: -100%;
transition: all 0.5s;
}
.nav-menu ul {
margin-top: 100px;
}
.nav-menu li {
margin-bottom: 30px;
}
.nav-menu a {
text-align: center;
font-size: 30px;
}
.checkbox:checked ~ .nav-menu {
left: 0;
}
main {
height: 1000px;
background: #CCFAFF;
}
<input type="checkbox" id="checkbox" class="checkbox">
と記述し、checkboxを表示します。
<label for="checkbox" class="hamburger">
<span class="bar bar-top"></span>
<span class="bar bar-middle"></span>
<span class="bar bar-bottom"></span>
</label>
作ったcheckboxに対してlabelタグを作成し、それをハンバーガーアイコンとして装飾していきます。
続いてクリックされたら表示されるナビメニューを作ります。
ナビメニューはcheckboxと同じ階層に記述します。
<nav class="nav-menu">
<ul>
<li><a href="#">menu1</a></li>
<li><a href="#">menu2</a></li>
<li><a href="#">menu3</a></li>
<li><a href="#">menu4</a></li>
</ul>
</nav>
と記述してあとはCSSで好みの形にデザインしていきます。
navタグのCSSには
position: fixed;
top: 0;
left: -100%;
position: fixed;で要素を浮かせた状態にしつつ、top: 0;とleft: -100%;で画面左端に隠した状態にしておきます。
続いてハンバーガーアイコンがクリックされた時にナビメニューが表示される仕組みを作ります。
先ほど作成したcheckboxにチェックが付いたか外れたかで判断するCSSを実装します。
.checkbox:checked ~ .nav-menu{
left: 0;
}
checkboxタグのクラス名に擬似クラス「:checked」を使用してチェックされた時だけ以下のプロパティを付け加えるようにします。
セレクターに「~ .nav-menu」と記述しているのは、checkboxタグの下の兄弟要素であるナビメニューに限定してプロパティを適用させるためです。
left: 0;で画面内に表示されるようにします。
最後に
.checkbox {
display: none;
}
とし、checkboxを非表示にすれば完成です。
JavaScriptを使ったハンバーガーメニュー
次にJavaScriptを使ったハンバーガーメニューの作り方も説明していきます。クリック判定に「onclick=””」属性を使っていきます。
<body>
<header>
<div class="logo">LOGO</div>
<div class="hamburger" id="hamburger" onclick="hamburgerClose()">
<span class="bar bar-top"></span>
<span class="bar bar-middle"></span>
<span class="bar bar-bottom"></span>
</div>
<nav class="nav-menu" id="nav-menu">
<ul>
<li><a href="#">menu1</a></li>
<li><a href="#">menu2</a></li>
<li><a href="#">menu3</a></li>
<li><a href="#">menu4</a></li>
</ul>
</nav>
</header>
<main></main>
<script src="/js/main.js"></script>
</body>
/* リセットCSS */
html, body, div, span, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
abbr, address, cite, code,
del, dfn, em, img, ins, kbd, q, samp,
small, strong, sub, sup, var,
b, i,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, figcaption, figure,
footer, header, hgroup, menu, nav, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
outline: 0;
font-size: 100%;
vertical-align: bottom;
background: transparent;
list-style: none;
}
body {
overflow-x: hidden;
}
*,
*::before,
*::after {
box-sizing: border-box;
}
a {
display: block;
color: inherit;
text-decoration: none;
font: inherit;
}
/* ここまでリセットCSS */
body {
font-family: "Arial", sans-serif;
color: #555;
font-feature-settings: "palt";
}
html {
scroll-behavior: smooth;
}
header {
position: fixed;
height: 80px;
width: 100%;
padding: 0 20px;
background: #fff;
display: flex;
justify-content: space-between;
align-items: center;
}
.logo {
font-size: 32px;
}
.hamburger {
width: 60px;
height: 60px;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
cursor: pointer;
}
.hamburger .bar {
width: 40px;
height: 3px;
background: #333;
border-radius: 2px;
display: block;
position: absolute;
transition: all 0.3s;
}
.hamburger .bar-top {
transform: translate(0, -12px);
}
.hamburger .bar-bottom {
transform: translate(0, 12px);
}
.hamburger.close .bar-middle {
opacity: 0;
}
.hamburger.close .bar-top {
transform: translate(0, 0) rotate(45deg);
}
.hamburger.close .bar-bottom {
transform: translate(0, 0) rotate(-45deg);
}
.nav-menu {
position: fixed;
width: 280px;
height: 100%;
background: #000;
opacity: 0.8;
color: #fff;
top: 0;
left: -100%;
transition: all 0.5s;
}
.nav-menu ul {
margin-top: 100px;
}
.nav-menu li {
margin-bottom: 30px;
}
.nav-menu a {
text-align: center;
font-size: 30px;
}
.nav-menu.close {
left: 0;
}
main {
height: 1000px;
background: #CCFAFF;
}
"use strict";
const hamburger = document.getElementById('hamburger');
const nav_menu = document.getElementById('nav-menu');
function hamburgerClose() {
hamburger.classList.toggle('close');
nav_menu.classList.toggle('close');
}
ポイントは
<div class="hamburger" id="hamburger" onclick="hamburgerClose()">
<!-- 省略 -->
</div>
に「onclick=””」属性を使い、JavaScriptの関数を呼びだしているところです。
HTMLのhamburger要素とnav-menu要素にそれぞれid名をつけ、JavaScriptのファイルに
const hamburger = document.getElementById('hamburger');
const nav_menu = document.getElementById('nav-menu');
とその要素を取得して定数を定義します。
続いてクリックされたら実行される関数を作成していきます。
function hamburgerClose() {
hamburger.classList.toggle('close');
nav_menu.classList.toggle('close');
}
「classList.toggle(‘close’)」でクリックされるごとにhamburger要素とnav-menu要素に「close」というclass名をつけたり外したりさせていきます。
cssで「close」が付いた時の処理を書きていきます。
.hamburger.close .bar-middle{
opacity: 0;
}
.hamburger.close .bar-top {
transform: translate(0,0) rotate(45deg);
}
.hamburger.close .bar-bottom {
transform: translate(0,0) rotate(-45deg);
}
.nav-menu.close{
left: 0;
}
セレクターは「.hamburger.close」や「.nav-menu.close」のようにスペースを空けないで繋げてかくのがポイントです。
レスポンシブに対応させたハンバーガーメニュー
ハンバーガーメニューは主に画面幅が狭いスマートフォンなどで使用されることがおおいです。
PCでは普通のナビゲーションメニューで表示し、スマートフォンでハンバーガーメニューに切り替えるようなレスポンシブに対応したサイトがユーザーの観点からみても観覧しやすいと思います。
レスポンシブに対応させたハンバーガーメニューの作り方を紹介します。
<body>
<header>
<div class="logo">LOGO</div>
<input type="checkbox" id="checkbox" class="checkbox">
<label for="checkbox" class="hamburger">
<span class="bar bar-top"></span>
<span class="bar bar-middle"></span>
<span class="bar bar-bottom"></span>
</label>
<nav class="nav-menu">
<ul>
<li><a href="#">menu1</a></li>
<li><a href="#">menu2</a></li>
<li><a href="#">menu3</a></li>
<li><a href="#">menu4</a></li>
</ul>
</nav>
</header>
<main></main>
</body>
html, body, div, span, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
abbr, address, cite, code,
del, dfn, em, img, ins, kbd, q, samp,
small, strong, sub, sup, var,
b, i,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, figcaption, figure,
footer, header, hgroup, menu, nav, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
outline: 0;
font-size: 100%;
vertical-align: bottom;
background: transparent;
list-style: none;
}
body {
overflow-x: hidden;
}
*,
*::before,
*::after {
box-sizing: border-box;
}
a {
display: block;
color: inherit;
text-decoration: none;
font: inherit;
}
body {
font-family: "Arial", sans-serif;
color: #555;
font-feature-settings: "palt";
}
html {
scroll-behavior: smooth;
}
header {
position: fixed;
height: 80px;
width: 100%;
padding: 0 20px;
background: #fff;
display: flex;
justify-content: space-between;
align-items: center;
}
.logo {
font-size: 32px;
}
.hamburger {
display: none;
width: 60px;
height: 60px;
justify-content: center;
align-items: center;
flex-direction: column;
cursor: pointer;
}
@media screen and (max-width: 700px) {
.hamburger {
display: flex;
}
}
.hamburger .bar {
width: 40px;
height: 3px;
background: #333;
border-radius: 2px;
display: block;
position: absolute;
transition: all 0.3s;
}
.hamburger .bar-top {
transform: translate(0, -12px);
}
.hamburger .bar-bottom {
transform: translate(0, 12px);
}
.checkbox:checked ~ .hamburger .bar-middle {
opacity: 0;
}
.checkbox:checked ~ .hamburger .bar-top {
transform: translate(0, 0) rotate(45deg);
}
.checkbox:checked ~ .hamburger .bar-bottom {
transform: translate(0, 0) rotate(-45deg);
}
.checkbox {
display: none;
}
.nav-menu {
position: static;
width: 500px;
}
@media screen and (max-width: 700px) {
.nav-menu {
position: fixed;
width: 280px;
height: 100%;
background: #000;
opacity: 0.8;
color: #fff;
top: 0;
left: -100%;
transition: all 0.5s;
}
}
.nav-menu ul {
display: flex;
justify-content: space-between;
}
@media screen and (max-width: 700px) {
.nav-menu ul {
margin-top: 100px;
display: block;
}
}
@media screen and (max-width: 700px) {
.nav-menu li {
margin-bottom: 30px;
}
}
.nav-menu a {
text-align: center;
font-size: 30px;
}
.checkbox:checked ~ .nav-menu {
left: 0;
}
main {
height: 1000px;
background: #CCFAFF;
}
ブラウザ幅が700px以下でハンバーガーメニューに切り替わるようになっています。
まずハンバーガーアイコンを700px以上で非表示、700px以下で表示するようにします。
.hamburger {
display: none;
width: 60px;
height: 60px;
justify-content: center;
align-items: center;
flex-direction: column;
cursor: pointer;
}
@media screen and (max-width: 700px) {
.hamburger {
display: flex;
}
}
「display: none;」であらかじめアイコンを非表示にしておき、
「@media screen and (max-width: 700px){}」と記述して700px以下のときに処理されるコードを{}内にかいていきます。
ここでは「display: flex;」と記述してアイコンを表示させました。
続いてナビメニューです。
.nav-menu {
width: 500px;
}
@media screen and (max-width: 700px) {
.nav-menu {
position: fixed;
width: 280px;
height: 100%;
background: #000;
opacity: 0.8;
color: #fff;
top: 0;
left: -100%;
transition: all 0.5s;
}
}
700px以下の時にスマートフォン向けのデザインに変更し、
「position: fixed;」と「left: -100%;」で画面外に隠しています。
ナビメニューの各アイテムを「display: flex;」であらかじめ横並びにしておき、
700px以下で縦並びに変更します。
.nav-menu ul {
display: flex;
justify-content: space-between;
}
@media screen and (max-width: 700px) {
.nav-menu ul {
margin-top: 100px;
display: block;
}
}
@media screen and (max-width: 700px) {
.nav-menu li {
margin-bottom: 30px;
}
}
以上、基本的なハンバーガーメニューのコードの書き方を説明しましたが、
もう少しアニメーションにこだわってみたいという時は以下のアニメーション一覧を参考にしてみてください。
メニューアイコンのアニメーション一覧
くるくる回る
See the Pen Untitled by teru (@teru241546) on CodePen.
真ん中に集まってから変わる
See the Pen Untitled by teru (@teru241546) on CodePen.
文字が切り替わる
See the Pen hamburger-icon-demo3 by teru (@teru241546) on CodePen.
メニューリストのアニメーション一覧
左からスッと現れるメニュー
<body>
<header>
<input type="checkbox" id="checkbox" class="checkbox">
<label for="checkbox" class="hamburger">
<span class="bar bar-top"></span>
<span class="bar bar-middle"></span>
<span class="bar bar-bottom"></span>
</label>
<nav class="nav-menu">
<ul>
<li><a href="#" data-number="1">menu1</a></li>
<li><a href="#" data-number="2">menu2</a></li>
<li><a href="#" data-number="3">menu3</a></li>
<li><a href="#" data-number="4">menu4</a></li>
</ul>
</nav>
</header>
</body>
/* リセットCSS */
html, body, div, span, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
abbr, address, cite, code,
del, dfn, em, img, ins, kbd, q, samp,
small, strong, sub, sup, var,
b, i,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, figcaption, figure,
footer, header, hgroup, menu, nav, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
outline: 0;
font-size: 100%;
vertical-align: bottom;
background: transparent;
list-style: none;
}
body {
overflow-x: hidden;
}
*,
*::before,
*::after {
box-sizing: border-box;
}
a {
display: block;
color: inherit;
text-decoration: none;
font: inherit;
}
body {
font-family: "Arial", sans-serif;
color: #555;
font-feature-settings: "palt";
}
html {
scroll-behavior: smooth;
}
/* ここまでリセットCSS */
header {
padding: 0 20px;
}
.hamburger {
width: 60px;
height: 72px;
justify-content: center;
align-items: center;
flex-direction: column;
cursor: pointer;
margin-left: auto;
display: flex;
transition: all 0.4s;
position: relative;
}
.checkbox:checked ~ .hamburger {
transform: rotate(360deg);
}
.hamburger .bar {
width: 40px;
height: 3px;
background: #333;
border-radius: 2px;
display: block;
position: absolute;
}
.hamburger .bar-top {
transform: translate(0, -12px) rotate(0);
transition: all 0.4s;
}
.hamburger .bar-middle {
transition: all 0.4s;
opacity: 1;
}
.hamburger .bar-bottom {
transform: translate(0, 12px) rotate(0);
transition: all 0.4s;
}
.checkbox:checked ~ .hamburger .bar-middle {
opacity: 0;
}
.checkbox:checked ~ .hamburger .bar-top {
transform: translate(0, 0) rotate(-45deg);
}
.checkbox:checked ~ .hamburger .bar-bottom {
transform: translate(0, 0) rotate(45deg);
}
.checkbox {
display: none;
}
.nav-menu {
position: fixed;
width: 280px;
height: 100%;
background: #000;
opacity: 0.8;
color: #fff;
top: 0;
left: -100%;
transition: all 0.5s;
}
.nav-menu ul {
margin-top: 160px;
position: relative;
}
.nav-menu li {
padding: 30px 0;
transform: translateX(-100%);
}
.nav-menu a {
text-align: center;
font-size: 30px;
}
.checkbox:checked ~ .nav-menu {
left: 0;
}
.checkbox:checked ~ .nav-menu li:nth-of-type(1) {
animation: nav-menu-li 1s 0.3s forwards;
}
.checkbox:checked ~ .nav-menu li:nth-of-type(2) {
animation: nav-menu-li 1s 0.6s forwards;
transition: all 0.4s 0.6s;
}
.checkbox:checked ~ .nav-menu li:nth-of-type(3) {
animation: nav-menu-li 1s 0.9s forwards;
transition: all 0.4s 0.9s;
}
.checkbox:checked ~ .nav-menu li:nth-of-type(4) {
animation: nav-menu-li 1s 1.2s forwards;
transition: all 0.4s 1.2s;
}
@keyframes nav-menu-li {
0% {
transform: translateX(-100%);
}
45% {
transform: translateX(4%);
}
70% {
transform: translateX(-2%);
}
100% {
transform: translateX(0);
}
}
背景がすりガラスのようになるメニュー
<body>
<header>
<input type="checkbox" id="checkbox" class="checkbox">
<label for="checkbox" class="hamburger">
<span class="bar bar-top"></span>
<span class="bar bar-middle"></span>
<span class="bar bar-bottom"></span>
</label>
<nav class="nav-menu">
<ul>
<li><a href="#" data-number="1">menu1</a></li>
<li><a href="#" data-number="2">menu2</a></li>
<li><a href="#" data-number="3">menu3</a></li>
<li><a href="#" data-number="4">menu4</a></li>
</ul>
</nav>
</header>
<main>
<p>テキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキスト</p>
<p>テキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキスト</p>
<p>テキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキストテキスト</p>
</main>
</body>
/* リセットCSS */
html, body, div, span, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
abbr, address, cite, code,
del, dfn, em, img, ins, kbd, q, samp,
small, strong, sub, sup, var,
b, i,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, figcaption, figure,
footer, header, hgroup, menu, nav, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
outline: 0;
font-size: 100%;
vertical-align: bottom;
background: transparent;
list-style: none;
}
body {
overflow-x: hidden;
}
*,
*::before,
*::after {
box-sizing: border-box;
}
a {
display: block;
color: inherit;
text-decoration: none;
font: inherit;
}
/* ここまでリセットCSS */
body {
font-family: "Arial", sans-serif;
color: #555;
font-feature-settings: "palt";
}
html {
scroll-behavior: smooth;
}
header {
padding: 0 20px;
}
.hamburger {
width: 60px;
height: 72px;
justify-content: center;
align-items: center;
flex-direction: column;
cursor: pointer;
margin-left: auto;
display: flex;
transition: all 0.4s;
position: relative;
z-index: 30;
}
.checkbox:checked ~ .hamburger {
transform: rotate(360deg);
}
.hamburger .bar {
width: 40px;
height: 3px;
background: #333;
border-radius: 2px;
display: block;
position: absolute;
}
.hamburger .bar-top {
transform: translate(0, -12px) rotate(0);
transition: all 0.4s;
}
.hamburger .bar-middle {
transition: all 0.4s;
opacity: 1;
}
.hamburger .bar-bottom {
transform: translate(0, 12px) rotate(0);
transition: all 0.4s;
}
.checkbox:checked ~ .hamburger .bar-middle {
opacity: 0;
}
.checkbox:checked ~ .hamburger .bar-top {
transform: translate(0, 0) rotate(-45deg);
}
.checkbox:checked ~ .hamburger .bar-bottom {
transform: translate(0, 0) rotate(45deg);
}
.checkbox {
display: none;
}
.nav-menu {
position: fixed;
width: 100%;
height: 100%;
opacity: 0;
color: #333;
top: 0;
left: 0;
transition: all 0.3s;
}
.nav-menu ul {
margin-top: 160px;
position: relative;
}
.nav-menu li {
padding: 30px 0;
}
.nav-menu a {
text-align: center;
font-size: 30px;
}
.checkbox:checked ~ .nav-menu {
backdrop-filter: blur(4px);
opacity: 1;
}
main p {
margin-bottom: 100px;
}
関連記事一覧
まだ投稿がありません。